Context Injection
Note: This feature is primarily designed to help you extract critical key-value pairs from a Slack alert and inject them into an investigation playbook that is executed during a workflow run.
A transformer function can be written (is equivalent of a lambda function) that will parse your alert and extract relevant key-value pairs from it. These key value pairs can then be injected within a playbook during it's run.
Instructions while writing a transformer function:
- Function must be named transform
- Input variable will be dictionary (in this case, it'll be the payload of Slack message)
- Output of the function must be a dictionary
- All import commands can be written within the function at it's start.
def transform(context):
import json
import re
out = {}
def extract_service_name(json_data):
# Convert JSON object to string
json_string = json.dumps(json_data)
# Search for the pattern "service:{service_name}"
match = re.search(r'service:(\w+)', json_string)
# If pattern is found, return the service name
if match:
return match.group(1)
else:
return ''
service_name = extract_service_name(context)
out['service_name'] = service_name
return out
def transform(context):
out = {}
for k in context.keys():
out[k] = str(context[k]) + "-a"
return out
Let's take a sample alert received:
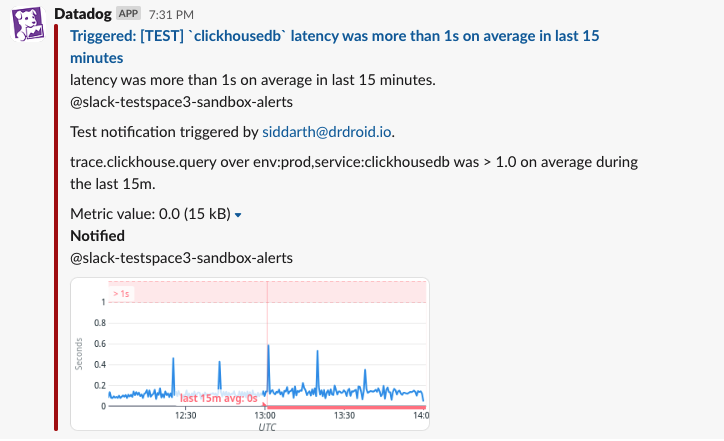
Here's the Slack JSON & it's output basis the above transform() function:
{
"event": {
"text": "",
"app_id": "AR28NTK5M",
"type": "message",
"metadata": {
"event_type": "monitor_thread",
"event_payload": {
"bits_monitor_info": {
"event_id": "7673797852050212359",
"is_enabled": false,
"hotdog": null
},
"event_id": 7673797852050213000,
"org_id": 945984,
"event_ts": 1721656861000,
"monitor_id": 141878267
}
},
"channel_type": "channel",
"event_ts": "1721656862.958529",
"attachments": [
{
"text": "latency was more than 1s on average in last 15 minutes.\n @slack-testspace3-sandbox-alerts\n\nTest notification triggered by <mailto:[email protected]|[email protected]>.\n\ntrace.clickhouse.query over env:prod,service:clickhousedb was > 1.0 on average during the last 15m.\n\nMetric value: 0.0",
"title_link": "https://app.datadoghq.com/monitors/141878267?from_ts=1721655960000&to_ts=1721657160000&event_id=7673797852050212359&source=monitor_notif",
"id": 1,
"fallback": "Triggered: [TEST] `clickhousedb` latency was more than 1s on average in last 15 minutes",
"image_height": 185,
"image_url": "https://p.datadoghq.com/snapshot/view/dd-snapshots-prod/org_945984/2024-07-22/91f3b20d9b9c36a8b9d283baddbd4e8e42d64563.png",
"fields": [
{
"title": "Notified",
"short": true,
"value": "@slack-testspace3-sandbox-alerts"
}
],
"title": "Triggered: [TEST] `clickhousedb` latency was more than 1s on average in last 15 minutes",
"mrkdwn_in": [
"fields",
"text"
],
"image_width": 431,
"color": "a30200",
"image_bytes": 15057
}
],
"bot_profile": {
"name": "Datadog",
"app_id": "AR28NTK5M",
"icons": {
"image_36": "https://avatars.slack-edge.com/2020-02-13/953695307206_a67bff8a85fff77da307_36.png",
"image_48": "https://avatars.slack-edge.com/2020-02-13/953695307206_a67bff8a85fff77da307_48.png",
"image_72": "https://avatars.slack-edge.com/2020-02-13/953695307206_a67bff8a85fff77da307_72.png"
},
"team_id": "T068A0EHN79",
"id": "B06AM27CLQ6",
"updated": 1712819434,
"deleted": false
},
"ts": "1721656862.958529",
"user": "U06AW4VR2DA",
"channel": "C076LUZQBC7",
"team": "T068A0EHN79",
"bot_id": "B06AM27CLQ6"
},
"type": "event_callback",
"api_app_id": "A071E0RUH71",
"is_ext_shared_channel": false,
"token": "Ei2euFvJ4yCyIVCYRD5AtpsK",
"event_time": 1721656862,
"event_context": "4-eyJldCI6Im1lc3NhZ2UiLCJ0aWQiOiJUMDY4QTBFSE43OSIsImFpZCI6IkEwNzFFMFJVSDcxIiwiY2lkIjoiQzA3NkxVWlFCQzcifQ",
"context_team_id": "T068A0EHN79",
"authorizations": [
{
"team_id": "T068A0EHN79",
"is_bot": true,
"user_id": "U072HB0GN00",
"enterprise_id": null,
"is_enterprise_install": false
}
],
"context_enterprise_id": null,
"event_id": "Ev07DGJMUZ98",
"team_id": "T068A0EHN79"
}
{
"service_name": "clickhousedb"
}
Updated about 1 month ago